Laughing
使用Hystrix实现微服务的熔断处理
Hystrix是Netflix开源的一个延迟和容错库,用于隔离访问远程系统、服务或者第三方库,防止级联失效,从而提升系统的可用性与容错性。
Hystrix基本使用
添加依赖
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
<version>2.2.8.RELEASE</version>
</dependency>
为启动类增加@EnableHystrix注解,从而为项目启用断路器支持
@SpringBootApplication
@EnableFeignClients
@EnableHystrix
public class MicroserviceConsumerMovieApplication {
public static void main(String[] args) {
SpringApplication.run(MicroserviceConsumerMovieApplication.class, args);
}
}
修改方法,增加容错能力
@GetMapping("/user/{id}")
@HystrixCommand(fallbackMethod = "findByIdFallback",
commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "5000"),
@HystrixProperty(name = "metrics.rollingStats.timeInMilliseconds", value = "10000")
},
threadPoolProperties = {
@HystrixProperty(name = "coreSize", value = "1"),
@HystrixProperty(name = "maxQueueSize", value = "10")
})
public User findById(@PathVariable Long id) {
return userFeignClient.findById(id);
}
public User findByIdFallback(Long id, Throwable throwable) {
log.info(throwable.getMessage());
User user = new User();
user.setName("默认用户");
return user;
}
测试
我们断开此时的服务提供者,访问方法,可以看到进入了容错方法,并获取到了异常信息。
feign集成hystrix
一定要注意Spring Cloud版本问题,Spring Cloud 2020 后,开启feign的hystrix支持需要在配置文件配置如下
feign:
circuitbreaker:
enabled: true
早期版本的Spring Cloud需要配置如下
feign:
hystrix:
enabled: true
为feign添加回退
通过fallback添加回退
通过fallback
添加回退,不能获取回退原因。
@FeignClient(name = "microservice-provider-user",fallback = UserFeignClientCallback.class)
public interface UserFeignClient {
/**
* 使用Feign自带的注解 @RequestLine
* @param id
* @return
*/
@RequestLine("GET /{id}")
User findById(@Param("id") Long id);
}
@Slf4j
@Component
class UserFeignClientCallback implements UserFeignClient{
/**
* 使用Feign自带的注解 @RequestLine
*
* @param id
* @return
*/
@Override
public User findById(Long id) {
User user = new User();
user.setName("默认用户");
return user;
}
}
通过fallbackFactory添加回退
fallbackFactory
是fallback
的升级版本,能够获取到回退原因。
@FeignClient(name = "microservice-provider-user",fallbackFactory = UserFeignClientCallbackFactory.class)
public interface UserFeignClient {
/**
* 使用Feign自带的注解 @RequestLine
* @param id
* @return
*/
@RequestLine("GET /{id}")
User findById(@Param("id") Long id);
}
@Slf4j
@Component
class UserFeignClientCallbackFactory implements FallbackFactory<UserFeignClient> {
@Override
public UserFeignClient create(Throwable cause) {
return new UserFeignClient() {
@Override
public User findById(Long id) {
log.info(cause.getMessage());
User user = new User();
user.setName("默认用户");
return user;
}
};
}
}
日志最好放到各个
fallback
方法中,而不要放到create
方法中,否则在引用启动时,会打印日志。feign项目中Hystrix的监控
首先需要添加依赖
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
<version>2.2.8.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
启动类添加@EnableHystrix
@SpringBootApplication
@EnableFeignClients
@EnableHystrix
public class MicroserviceConsumerMovieApplication {
public static void main(String[] args) {
SpringApplication.run(MicroserviceConsumerMovieApplication.class, args);
}
}
修改配置文件,暴漏地址
management:
endpoints:
web:
exposure:
include: hystrix.stream
测试
打开地址http://localhost:8081/actuator/hystrix.stream
使用hystrix-dashboard可视化监控项目
我们刚才项目监控时,返回的都是Json数据,通过hystrix-dashboard我们可以实现可视化的监控。
添加hystrix-dashboard依赖
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix-dashboard</artifactId>
<version>2.2.8.RELEASE</version>
</dependency>
在启动类添加@EnableHystrixDashboard注解
@SpringBootApplication
@EnableFeignClients
@EnableHystrix
@EnableHystrixDashboard
public class MicroserviceConsumerMovieApplication {
public static void main(String[] args) {
SpringApplication.run(MicroserviceConsumerMovieApplication.class, args);
}
}
修改配置文件
配置文件添加
hystrix:
dashboard:
proxy-stream-allow-list: "*"
如果不添加,监控会提示Unable to connect to Command Metric Stream.
测试
打开http://localhost:8081/hystrix
,监控地址输入http://localhost:8081/actuator/hystrix.stream
,title随便输入即可。
点击Monitor Stream
,然后访问任意的接口,系统输出界面如下
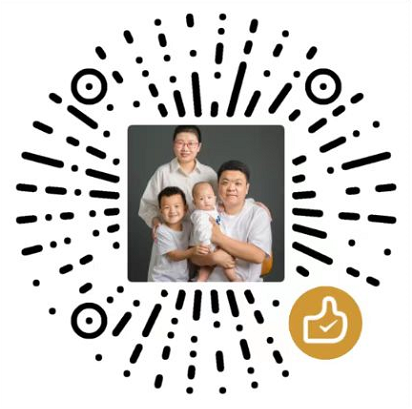
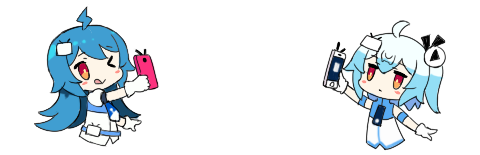
香草物语
https://www.xiangcaowuyu.net/java/fuse-processing-of-microservices-using-hystrix.html(转载时请注明本文出处及文章链接)