Laughing
Spring Boot分开打包依赖及配置文件
壹、为何要分开打包依赖
Spring Boot默认会将依赖全部打包到一个jar中,这样导致的问题就是我们的一个jar往往很大。加之平时我们分模块开发,往往修改很小的一个部分,就需要打包整个jar包,上传整个jar到服务器。比如我用阿里云服务器,3M的带宽,如果我不拆分开依赖,仅仅是上传jar都需要耗时接近1分钟的时间。
当然这样也有一些其他问题,比如我这种多模块的项目,如果我们修改了其他模块(非启动类所在模块),那么我们需要记得将打包的jar要放到依赖对应的文件夹中。
贰、为何要分开打包配置文件
相对于分开打包依赖,其实配置文件才是更有必要打包的。Spring Boot配置文件默认包裹在jar包中的形式,一方面容易造成配置文件的覆盖,另一方面修改配置文件也相对比较麻烦。
叁、如何拆分打包依赖及配置文件
Spring Boot分开打包依赖及配置文件的方法也比较简单,我们只需要修改pom.xml
文件即可。
只需要注意一点就是,如果我们是多模块的项目,需要修改主工程的pom.xml
文件。
添加一些配置属性,方便修改
<properties>
<!--依赖输出目录-->
<output.dependence.file.path>../output/lib/</output.dependence.file.path>
<!--manifest中lib配置路径-->
<manifest.classpath.prefix>lib</manifest.classpath.prefix>
<!--jar输出目录-->
<output.jar.file.path>../output/</output.jar.file.path>
<!--配置文件输出目录-->
<output.resource.file.path>../output/config/</output.resource.file.path>
</properties>
我这里实现的效果是把所有的文件都放到项目顶级的output
文件夹中,项目的jar放到output
中,依赖放到lib
文件夹中,配置文件放到config
文件夹中
然后我们修改打包插件
<build>
<plugins>
<!-- 打JAR包,不包含依赖文件;显式剔除配置文件 -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<outputDirectory>${output.jar.file.path}</outputDirectory>
<!-- 将配置文件排除在jar包 -->
<excludes>
<exclude>*.properties</exclude>
<exclude>*.yml</exclude>
<exclude>*.xml</exclude>
<exclude>*.txt</exclude>
</excludes>
<archive>
<!-- 生成的jar中,包含pom.xml和pom.properties这两个文件 -->
<addMavenDescriptor>true</addMavenDescriptor>
<!-- 生成MANIFEST.MF的设置 -->
<manifest>
<!--这个属性特别关键,如果没有这个属性,有时候我们引用的包maven库 下面可能会有多个包,并且只有一个是正确的,
其余的可能是带时间戳的,此时会在classpath下面把那个带时间戳的给添加上去,然后我们 在依赖打包的时候,
打的是正确的,所以两头会对不上,报错。 -->
<useUniqueVersions>false</useUniqueVersions>
<!-- 为依赖包添加路径, 这些路径会写在MANIFEST文件的Class-Path下 -->
<addClasspath>true</addClasspath>
<!-- MANIFEST.MF 中 Class-Path 各个依赖加入前缀 -->
<!--这个jar所依赖的jar包添加classPath的时候的前缀,需要 下面maven-dependency-plugin插件补充-->
<!--一定要找对目录,否则jar找不到依赖lib-->
<classpathPrefix>${manifest.classpath.prefix}</classpathPrefix>
<!--指定jar启动入口类 -->
<mainClass>net.xiangcaowuyu.LeeFrameApplication</mainClass>
</manifest>
<manifestEntries>
<!-- 假如这个项目可能要引入一些外部资源,但是你打包的时候并不想把 这些资源文件打进包里面,这个时候你必须在
这边额外指定一些这些资源文件的路径,假如你的pom文件里面配置了 <scope>system</scope>,就是你依赖是你本地的
资源,这个时候使用这个插件,classPath里面是不会添加,所以你得手动把这个依赖添加进这个地方 -->
<!--MANIFEST.MF 中 Class-Path 加入自定义路径,多个路径用空格隔开 -->
<!--此处resources文件夹的内容,需要maven-resources-plugin插件补充上-->
<Class-Path>${output.resource.file.path}</Class-Path>
</manifestEntries>
</archive>
</configuration>
</plugin>
<!-- 复制依赖的jar包到指定的文件夹里 -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<executions>
<execution>
<id>copy-dependencies</id>
<phase>package</phase>
<goals>
<goal>copy-dependencies</goal>
</goals>
<configuration>
<!-- 拷贝项目依赖包到指定目录下 -->
<outputDirectory>${output.dependence.file.path}</outputDirectory>
<!-- 是否排除间接依赖,间接依赖也要拷贝 -->
<excludeTransitive>false</excludeTransitive>
<!-- 是否带上版本号 -->
<stripVersion>false</stripVersion>
</configuration>
</execution>
</executions>
</plugin>
<!-- 用于复制指定的文件 -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<executions>
<!-- 复制配置文件 -->
<execution>
<id>copy-resources</id>
<phase>package</phase>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<resources>
<resource>
<directory>src/main/resources</directory>
<includes>
<!--将如下格式配置文件拷贝-->
<exclude>*.properties</exclude>
<exclude>*.yml</exclude>
<exclude>*.xml</exclude>
<exclude>*.txt</exclude>
</includes>
</resource>
</resources>
<!--输出路径-->
<outputDirectory>${output.resource.file.path}</outputDirectory>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
<finalName>${project.artifactId}</finalName>
</build>
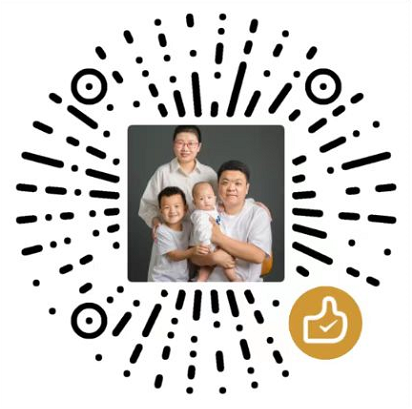
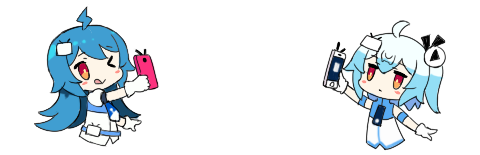
香草物语
https://www.xiangcaowuyu.net/java/spring-boot-packages-dependencies-and-configuration-files-separately.html(转载时请注明本文出处及文章链接)